Qt Signal Slot Infinite Loop
The QTimer class provides repetitive and single-shot timers. More..
- Qt Signals And Slots Tutorial
- Qt Signal Slot With 2 Arguments
- Signal Qt
- Qt Signal Slot Infinite Loop Free
- Qt Signal Slot Connect
- Qt Signal Slot Infinite Loop 3
Here, the signal is emitted in the worker thread, and the slot is executed in the GUI thread when control returns to the event loop. With queued connections, Qt must store a copy of the arguments that were passed to the signal so that it can pass them to the slot later on. Connecting in Qt 5. There are several ways to connect a signal in Qt 5. Qt 5 continues to support the old string-based syntax for connecting signals and slots defined in a QObject or any class that inherits from QObject (including QWidget). Connect( sender, SIGNAL( valueChanged( QString, QString ) ), receiver, SLOT( updateValue( QString ) ) ). If you get a signal for each packet (e.g. By the QNetwork. classes), then you could just forget about the loop. Just connect some handler to the signal, and have it update the QTableWidget whenever it gets the signal. The 'infinite looping' is then handled by the event loop inside QApplication.
Header: | #include <QTimer> |
qmake: | QT += core |
Inherits: | QObject |
Properties
|
|
Public Functions
QTimer(QObject *parent = nullptr) | |
virtual | ~QTimer() |
QMetaObject::Connection | callOnTimeout(Functor slot, Qt::ConnectionType connectionType = ..) |
QMetaObject::Connection | callOnTimeout(const QObject *context, Functor slot, Qt::ConnectionType connectionType = ..) |
QMetaObject::Connection | callOnTimeout(const QObject *receiver, MemberFunction *slot, Qt::ConnectionType connectionType = ..) |
int | interval() const |
std::chrono::milliseconds | intervalAsDuration() const |
bool | isActive() const |
bool | isSingleShot() const |
int | remainingTime() const |
std::chrono::milliseconds | remainingTimeAsDuration() const |
void | setInterval(int msec) |
void | setInterval(std::chrono::milliseconds value) |
void | setSingleShot(bool singleShot) |
void | setTimerType(Qt::TimerType atype) |
void | start(std::chrono::milliseconds msec) |
int | timerId() const |
Qt::TimerType | timerType() const |
Public Slots
Signals
void | timeout() |
Static Public Members
void | singleShot(int msec, const QObject *receiver, const char *member) |
void | singleShot(int msec, Qt::TimerType timerType, const QObject *receiver, const char *member) |
void | singleShot(int msec, const QObject *receiver, PointerToMemberFunction method) |
void | singleShot(int msec, Qt::TimerType timerType, const QObject *receiver, PointerToMemberFunction method) |
void | singleShot(int msec, Functor functor) |
void | singleShot(int msec, Qt::TimerType timerType, Functor functor) |
void | singleShot(int msec, const QObject *context, Functor functor) |
void | singleShot(int msec, Qt::TimerType timerType, const QObject *context, Functor functor) |
void | singleShot(std::chrono::milliseconds msec, const QObject *receiver, const char *member) |
void | singleShot(std::chrono::milliseconds msec, Qt::TimerType timerType, const QObject *receiver, const char *member) |
Reimplemented Protected Functions
Detailed Description
The QTimer class provides a high-level programming interface for timers. To use it, create a QTimer, connect its timeout() signal to the appropriate slots, and call start(). From then on, it will emit the timeout() signal at constant intervals.
Example for a one second (1000 millisecond) timer (from the Analog Clock example):
From then on, the update()
slot is called every second.
You can set a timer to time out only once by calling setSingleShot(true). You can also use the static QTimer::singleShot() function to call a slot after a specified interval:
In multithreaded applications, you can use QTimer in any thread that has an event loop. To start an event loop from a non-GUI thread, use QThread::exec(). Qt uses the timer's thread affinity to determine which thread will emit the timeout() signal. Because of this, you must start and stop the timer in its thread; it is not possible to start a timer from another thread.
As a special case, a QTimer with a timeout of 0 will time out as soon as possible, though the ordering between zero timers and other sources of events is unspecified. Zero timers can be used to do some work while still providing a snappy user interface:
From then on, processOneThing()
will be called repeatedly. It should be written in such a way that it always returns quickly (typically after processing one data item) so that Qt can deliver events to the user interface and stop the timer as soon as it has done all its work. This is the traditional way of implementing heavy work in GUI applications, but as multithreading is nowadays becoming available on more and more platforms, we expect that zero-millisecond QTimer objects will gradually be replaced by QThreads.
Accuracy and Timer Resolution
The accuracy of timers depends on the underlying operating system and hardware. Most platforms support a resolution of 1 millisecond, though the accuracy of the timer will not equal this resolution in many real-world situations.
The accuracy also depends on the timer type. For Qt::PreciseTimer, QTimer will try to keep the accuracy at 1 millisecond. Precise timers will also never time out earlier than expected.
For Qt::CoarseTimer and Qt::VeryCoarseTimer types, QTimer may wake up earlier than expected, within the margins for those types: 5% of the interval for Qt::CoarseTimer and 500 ms for Qt::VeryCoarseTimer.
All timer types may time out later than expected if the system is busy or unable to provide the requested accuracy. In such a case of timeout overrun, Qt will emit timeout() only once, even if multiple timeouts have expired, and then will resume the original interval.
Alternatives to QTimer
An alternative to using QTimer is to call QObject::startTimer() for your object and reimplement the QObject::timerEvent() event handler in your class (which must inherit QObject). The disadvantage is that timerEvent() does not support such high-level features as single-shot timers or signals.
Another alternative is QBasicTimer. It is typically less cumbersome than using QObject::startTimer() directly. See Timers for an overview of all three approaches.
Some operating systems limit the number of timers that may be used; Qt tries to work around these limitations.
See also QBasicTimer, QTimerEvent, QObject::timerEvent(), Timers, Analog Clock Example, and Wiggly Example.
Property Documentation
active : const bool
This boolean property is true
if the timer is running; otherwise false.
This property was introduced in Qt 4.3.
Access functions:
interval : int
This property holds the timeout interval in milliseconds
The default value for this property is 0. A QTimer with a timeout interval of 0 will time out as soon as all the events in the window system's event queue have been processed.
Setting the interval of an active timer changes its timerId().
Access functions:
int | interval() const |
void | setInterval(int msec) |
void | setInterval(std::chrono::milliseconds value) |
See also singleShot.
remainingTime : const int
This property holds the remaining time in milliseconds
Returns the timer's remaining value in milliseconds left until the timeout. If the timer is inactive, the returned value will be -1. If the timer is overdue, the returned value will be 0.
This property was introduced in Qt 5.0.
Access functions:
See also interval.
singleShot : bool
This property holds whether the timer is a single-shot timer
A single-shot timer fires only once, non-single-shot timers fire every interval milliseconds.
The default value for this property is false
.
Access functions:
bool | isSingleShot() const |
void | setSingleShot(bool singleShot) |
See also interval and singleShot().
timerType : Qt::TimerType
controls the accuracy of the timer
The default value for this property is Qt::CoarseTimer
.
Access functions:
Qt::TimerType | timerType() const |
void | setTimerType(Qt::TimerType atype) |
See also Qt::TimerType.
Member Function Documentation
QTimer::QTimer(QObject *parent = nullptr)
Constructs a timer with the given parent.
[slot]
void QTimer::start()
This function overloads start().
Starts or restarts the timer with the timeout specified in interval.
If the timer is already running, it will be stopped and restarted.
If singleShot is true, the timer will be activated only once.
[slot]
void QTimer::start(intmsec)
Starts or restarts the timer with a timeout interval of msec milliseconds.
If the timer is already running, it will be stopped and restarted.
If singleShot is true, the timer will be activated only once.
[slot]
void QTimer::stop()
Stops the timer.
See also start().
[signal]
void QTimer::timeout()
This signal is emitted when the timer times out.
Note: This is a private signal. It can be used in signal connections but cannot be emitted by the user.
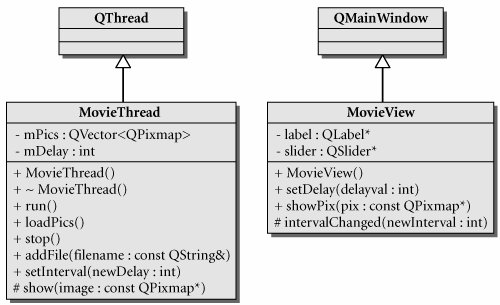
See also interval, start(), and stop().
[virtual]
QTimer::~QTimer()
Destroys the timer.
QMetaObject::Connection QTimer::callOnTimeout(Functorslot, Qt::ConnectionTypeconnectionType = ..)
This is an overloaded function.
Creates a connection of type connectionType from the timeout() signal to slot, and returns a handle to the connection.
This method is provided for convenience. It's equivalent to calling QObject::connect(timer, &QTimer::timeout, timer, slot, connectionType)
.
This function was introduced in Qt 5.12.
See also QObject::connect() and timeout().
QMetaObject::Connection QTimer::callOnTimeout(const QObject *context, Functorslot, Qt::ConnectionTypeconnectionType = ..)
This function overloads callOnTimeout().
Creates a connection from the timeout() signal to slot to be placed in a specific event loop of context, and returns a handle to the connection.
This method is provided for convenience. It's equivalent to calling QObject::connect(timer, &QTimer::timeout, context, slot, connectionType)
.
This function was introduced in Qt 5.12.
See also QObject::connect() and timeout().
QMetaObject::Connection QTimer::callOnTimeout(const QObject *receiver, MemberFunction *slot, Qt::ConnectionTypeconnectionType = ..)
This function overloads callOnTimeout().
Creates a connection from the timeout() signal to the slot in the receiver object. Returns a handle to the connection.
This method is provided for convenience. It's equivalent to calling QObject::connect(timer, &QTimer::timeout, receiver, slot, connectionType)
.
This function was introduced in Qt 5.12.
See also QObject::connect() and timeout().
std::chrono::milliseconds QTimer::intervalAsDuration() const
Returns the interval of this timer as a std::chrono::milliseconds
object.
This function was introduced in Qt 5.8.
See also interval.
bool QTimer::isActive() const
Returns true
if the timer is running (pending); otherwise returns false.
Note: Getter function for property active.
std::chrono::milliseconds QTimer::remainingTimeAsDuration() const
Returns the time remaining in this timer object as a std::chrono::milliseconds
object. If this timer is due or overdue, the returned value is std::chrono::milliseconds::zero()
. If the remaining time could not be found or the timer is not active, this function returns a negative duration.
This function was introduced in Qt 5.8.
See also remainingTime().
[static]
void QTimer::singleShot(intmsec, const QObject *receiver, const char *member)
This static function calls a slot after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent or create a local QTimer object.
Example:
This sample program automatically terminates after 10 minutes (600,000 milliseconds).
The receiver is the receiving object and the member is the slot. The time interval is msec milliseconds.
Note: This function is reentrant.
See also setSingleShot() and start().
[static]
void QTimer::singleShot(intmsec, Qt::TimerTypetimerType, const QObject *receiver, const char *member)
This is an overloaded function. Wild wolf slot casino games.
This static function calls a slot after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent or create a local QTimer object.
The receiver is the receiving object and the member is the slot. The time interval is msec milliseconds. The timerType affects the accuracy of the timer.
Note: This function is reentrant.
See also start().
[static]
void QTimer::singleShot(intmsec, const QObject *receiver, PointerToMemberFunctionmethod)
This is an overloaded function.
This static function calls a member function of a QObject after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent or create a local QTimer object.
The receiver is the receiving object and the method is the member function. The time interval is msec milliseconds.
If receiver is destroyed before the interval occurs, the method will not be called. The function will be run in the thread of receiver. The receiver's thread must have a running Qt event loop.
Note: This function is reentrant.
This function was introduced in Qt 5.4.
See also start().
[static]
void QTimer::singleShot(intmsec, Qt::TimerTypetimerType, const QObject *receiver, PointerToMemberFunctionmethod)
This is an overloaded function.
This static function calls a member function of a QObject after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent or create a local QTimer object.
The receiver is the receiving object and the method is the member function. The time interval is msec milliseconds. The timerType affects the accuracy of the timer.
If receiver is destroyed before the interval occurs, the method will not be called. The function will be run in the thread of receiver. The receiver's thread must have a running Qt event loop.
Note: This function is reentrant.
This function was introduced in Qt 5.4.
See also start().
[static]
void QTimer::singleShot(intmsec, Functorfunctor)
This is an overloaded function.
This static function calls functor after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent or create a local QTimer object.
The time interval is msec milliseconds.
Note: This function is reentrant.
This function was introduced in Qt 5.4.
See also start().
[static]
void QTimer::singleShot(intmsec, Qt::TimerTypetimerType, Functorfunctor)
This is an overloaded function.
This static function calls functor after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent or create a local QTimer object.
The time interval is msec milliseconds. The timerType affects the accuracy of the timer.
Note: This function is reentrant.
This function was introduced in Qt 5.4.
See also start().
[static]
void QTimer::singleShot(intmsec, const QObject *context, Functorfunctor)
This is an overloaded function.
This static function calls functor after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent or create a local QTimer object.
The time interval is msec milliseconds.
If context is destroyed before the interval occurs, the method will not be called. The function will be run in the thread of context. The context's thread must have a running Qt event loop.
Note: This function is reentrant.
This function was introduced in Qt 5.4.
See also start().
[static]
void QTimer::singleShot(intmsec, Qt::TimerTypetimerType, const QObject *context, Functorfunctor)
This is an overloaded function.
This static function calls functor after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent or create a local QTimer object.
The time interval is msec milliseconds. The timerType affects the accuracy of the timer.
If context is destroyed before the interval occurs, the method will not be called. The function will be run in the thread of context. The context's thread must have a running Qt event loop.
Note: This function is reentrant.
This function was introduced in Qt 5.4.
See also start().
[static]
void QTimer::singleShot(std::chrono::millisecondsmsec, const QObject *receiver, const char *member)
This is an overloaded function.
This static function calls a slot after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent or create a local QTimer object.
The receiver is the receiving object and the member is the slot. The time interval is given in the duration object msec.
Note: This function is reentrant.
This function was introduced in Qt 5.8.
See also start().
[static]
void QTimer::singleShot(std::chrono::millisecondsmsec, Qt::TimerTypetimerType, const QObject *receiver, const char *member)
This is an overloaded function.
This static function calls a slot after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent or create a local QTimer object.
The receiver is the receiving object and the member is the slot. The time interval is given in the duration object msec. The timerType affects the accuracy of the timer.
Note: This function is reentrant.
This function was introduced in Qt 5.8.
See also start().
void QTimer::start(std::chrono::millisecondsmsec)
This is an overloaded function.
Starts or restarts the timer with a timeout of duration msec milliseconds.
If the timer is already running, it will be stopped and restarted.
If singleShot is true, the timer will be activated only once.
This function was introduced in Qt 5.8.
[override virtual protected]
void QTimer::timerEvent(QTimerEvent *e)
Reimplements: QObject::timerEvent(QTimerEvent *event).
int QTimer::timerId() const
Returns the ID of the timer if the timer is running; otherwise returns -1.
© 2020 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.
An overview of Qt’s signals and slots inter-object communication mechanism.
Signals and slots are used for communication between objects. The signals and slots mechanism is a central feature of Qt and probably the part that differs most from the features provided by other frameworks. Signals and slots are made possible by Qt’s meta-object system .
Introduction¶
In GUI programming, when we change one widget, we often want another widget to be notified. More generally, we want objects of any kind to be able to communicate with one another. For example, if a user clicks a Close button, we probably want the window’s close()
function to be called.
Other toolkits achieve this kind of communication using callbacks. A callback is a pointer to a function, so if you want a processing function to notify you about some event you pass a pointer to another function (the callback) to the processing function. The processing function then calls the callback when appropriate. While successful frameworks using this method do exist, callbacks can be unintuitive and may suffer from problems in ensuring the type-correctness of callback arguments.
Signals and Slots¶
In Qt, we have an alternative to the callback technique: We use signals and slots. A signal is emitted when a particular event occurs. Qt’s widgets have many predefined signals, but we can always subclass widgets to add our own signals to them. A slot is a function that is called in response to a particular signal. Qt’s widgets have many pre-defined slots, but it is common practice to subclass widgets and add your own slots so that you can handle the signals that you are interested in.
The signals and slots mechanism is type safe: The signature of a signal must match the signature of the receiving slot. (In fact a slot may have a shorter signature than the signal it receives because it can ignore extra arguments.) Since the signatures are compatible, the compiler can help us detect type mismatches when using the function pointer-based syntax. The string-based SIGNAL and SLOT syntax will detect type mismatches at runtime. Signals and slots are loosely coupled: A class which emits a signal neither knows nor cares which slots receive the signal. Qt’s signals and slots mechanism ensures that if you connect a signal to a slot, the slot will be called with the signal’s parameters at the right time. Signals and slots can take any number of arguments of any type. They are completely type safe.
All classes that inherit from QObject
or one of its subclasses (e.g., QWidget
) can contain signals and slots. Signals are emitted by objects when they change their state in a way that may be interesting to other objects. This is all the object does to communicate. It does not know or care whether anything is receiving the signals it emits. This is true information encapsulation, and ensures that the object can be used as a software component.
Slots can be used for receiving signals, but they are also normal member functions. Just as an object does not know if anything receives its signals, a slot does not know if it has any signals connected to it. This ensures that truly independent components can be created with Qt.
You can connect as many signals as you want to a single slot, and a signal can be connected to as many slots as you need. It is even possible to connect a signal directly to another signal. (This will emit the second signal immediately whenever the first is emitted.)
Together, signals and slots make up a powerful component programming mechanism.
Signals¶
Signals are emitted by an object when its internal state has changed in some way that might be interesting to the object’s client or owner. Signals are public access functions and can be emitted from anywhere, but we recommend to only emit them from the class that defines the signal and its subclasses.
When a signal is emitted, the slots connected to it are usually executed immediately, just like a normal function call. When this happens, the signals and slots mechanism is totally independent of any GUI event loop. Execution of the code following the emit
statement will occur once all slots have returned. The situation is slightly different when using queuedconnections
; in such a case, the code following the emit
keyword will continue immediately, and the slots will be executed later.
If several slots are connected to one signal, the slots will be executed one after the other, in the order they have been connected, when the signal is emitted.
Signals are automatically generated by the moc and must not be implemented in the .cpp
file. They can never have return types (i.e. use void
).
A note about arguments: Our experience shows that signals and slots are more reusable if they do not use special types. If valueChanged()
were to use a special type such as the hypothetical QScrollBar::Range, it could only be connected to slots designed specifically for QScrollBar
. Connecting different input widgets together would be impossible.
Slots¶
A slot is called when a signal connected to it is emitted. Slots are normal C++ functions and can be called normally; their only special feature is that signals can be connected to them.
Since slots are normal member functions, they follow the normal C++ rules when called directly. However, as slots, they can be invoked by any component, regardless of its access level, via a signal-slot connection. This means that a signal emitted from an instance of an arbitrary class can cause a private slot to be invoked in an instance of an unrelated class.
You can also define slots to be virtual, which we have found quite useful in practice.
Compared to callbacks, signals and slots are slightly slower because of the increased flexibility they provide, although the difference for real applications is insignificant. In general, emitting a signal that is connected to some slots, is approximately ten times slower than calling the receivers directly, with non-virtual function calls. This is the overhead required to locate the connection object, to safely iterate over all connections (i.e. checking that subsequent receivers have not been destroyed during the emission), and to marshall any parameters in a generic fashion. While ten non-virtual function calls may sound like a lot, it’s much less overhead than any new
or delete
operation, for example. As soon as you perform a string, vector or list operation that behind the scene requires new
or delete
, the signals and slots overhead is only responsible for a very small proportion of the complete function call costs. The same is true whenever you do a system call in a slot; or indirectly call more than ten functions. The simplicity and flexibility of the signals and slots mechanism is well worth the overhead, which your users won’t even notice.
Note that other libraries that define variables called signals
or slots
may cause compiler warnings and errors when compiled alongside a Qt-based application. To solve this problem, #undef
the offending preprocessor symbol.
A Small Example¶
A minimal C++ class declaration might read:
A small QObject
-based class might read:
The QObject
-based version has the same internal state, and provides public methods to access the state, but in addition it has support for component programming using signals and slots. This class can tell the outside world that its state has changed by emitting a signal, valueChanged()
, and it has a slot which other objects can send signals to.
All classes that contain signals or slots must mention Q_OBJECT
at the top of their declaration. They must also derive (directly or indirectly) from QObject
.
Qt Signals And Slots Tutorial
Slots are implemented by the application programmer. Here is a possible implementation of the Counter::setValue()
slot:
The emit
line emits the signal valueChanged()
from the object, with the new value as argument.
In the following code snippet, we create two Counter
objects and connect the first object’s valueChanged()
signal to the second object’s setValue()
slot using connect()
:
Calling a.setValue(12)
makes a
emit a valueChanged(12)
signal, which b
will receive in its setValue()
slot, i.e. b.setValue(12)
is called. Then b
emits the same valueChanged()
signal, but since no slot has been connected to b
‘s valueChanged()
signal, the signal is ignored.
Note that the setValue()
function sets the value and emits the signal only if value!=m_value
. This prevents infinite looping in the case of cyclic connections (e.g., if b.valueChanged()
were connected to a.setValue()
).
By default, for every connection you make, a signal is emitted; two signals are emitted for duplicate connections. You can break all of these connections with a single disconnect()
call. If you pass the UniqueConnection
type
, the connection will only be made if it is not a duplicate. If there is already a duplicate (exact same signal to the exact same slot on the same objects), the connection will fail and connect will return false
.
This example illustrates that objects can work together without needing to know any information about each other. To enable this, the objects only need to be connected together, and this can be achieved with some simple connect()
function calls, or with uic’s automatic connections feature.
A Real Example¶
The following is an example of the header of a simple widget class without member functions. The purpose is to show how you can utilize signals and slots in your own applications.
LcdNumber
inherits QObject
, which has most of the signal-slot knowledge, via QFrame
and QWidget
. It is somewhat similar to the built-in QLCDNumber
widget.
Qt Signal Slot With 2 Arguments
The Q_OBJECT
macro is expanded by the preprocessor to declare several member functions that are implemented by the moc
; if you get compiler errors along the lines of “undefined reference to vtable for LcdNumber
“, you have probably forgotten to run the moc or to include the moc output in the link command.
After the class constructor and public
members, we declare the class signals
. The LcdNumber
class emits a signal, overflow()
, when it is asked to show an impossible value.
If you don’t care about overflow, or you know that overflow cannot occur, you can ignore the overflow()
signal, i.e. don’t connect it to any slot.
If on the other hand you want to call two different error functions when the number overflows, simply connect the signal to two different slots. Qt will call both (in the order they were connected).
A slot is a receiving function used to get information about state changes in other widgets. LcdNumber
uses it, as the code above indicates, to set the displayed number. Since display()
is part of the class’s interface with the rest of the program, the slot is public.
Several of the example programs connect the valueChanged()
signal of a QScrollBar
to the display()
slot, so the LCD number continuously shows the value of the scroll bar.
Note that display()
is overloaded; Qt will select the appropriate version when you connect a signal to the slot. With callbacks, you’d have to find five different names and keep track of the types yourself.
Signals And Slots With Default Arguments¶
The signatures of signals and slots may contain arguments, and the arguments can have default values. Consider destroyed()
:
When a QObject
is deleted, it emits this destroyed()
signal. We want to catch this signal, wherever we might have a dangling reference to the deleted QObject
, so we can clean it up. A suitable slot signature might be:
To connect the signal to the slot, we use connect()
. There are several ways to connect signal and slots. The first is to use function pointers:
Signal Qt
There are several advantages to using connect()
with function pointers. First, it allows the compiler to check that the signal’s arguments are compatible with the slot’s arguments. Arguments can also be implicitly converted by the compiler, if needed.
You can also connect to functors or C++11 lambdas:
In both these cases, we provide this
as context in the call to connect(). The context object provides information about in which thread the receiver should be executed. This is important, as providing the context ensures that the receiver is executed in the context thread.
The lambda will be disconnected when the sender or context is destroyed. You should take care that any objects used inside the functor are still alive when the signal is emitted.
The other way to connect a signal to a slot is to use connect()
and the SIGNAL
and SLOT
macros. The rule about whether to include arguments or not in the SIGNAL()
and SLOT()
macros, if the arguments have default values, is that the signature passed to the SIGNAL()
macro must not have fewer arguments than the signature passed to the SLOT()
macro.
Qt Signal Slot Infinite Loop Free
All of these would work:
But this one won’t work:
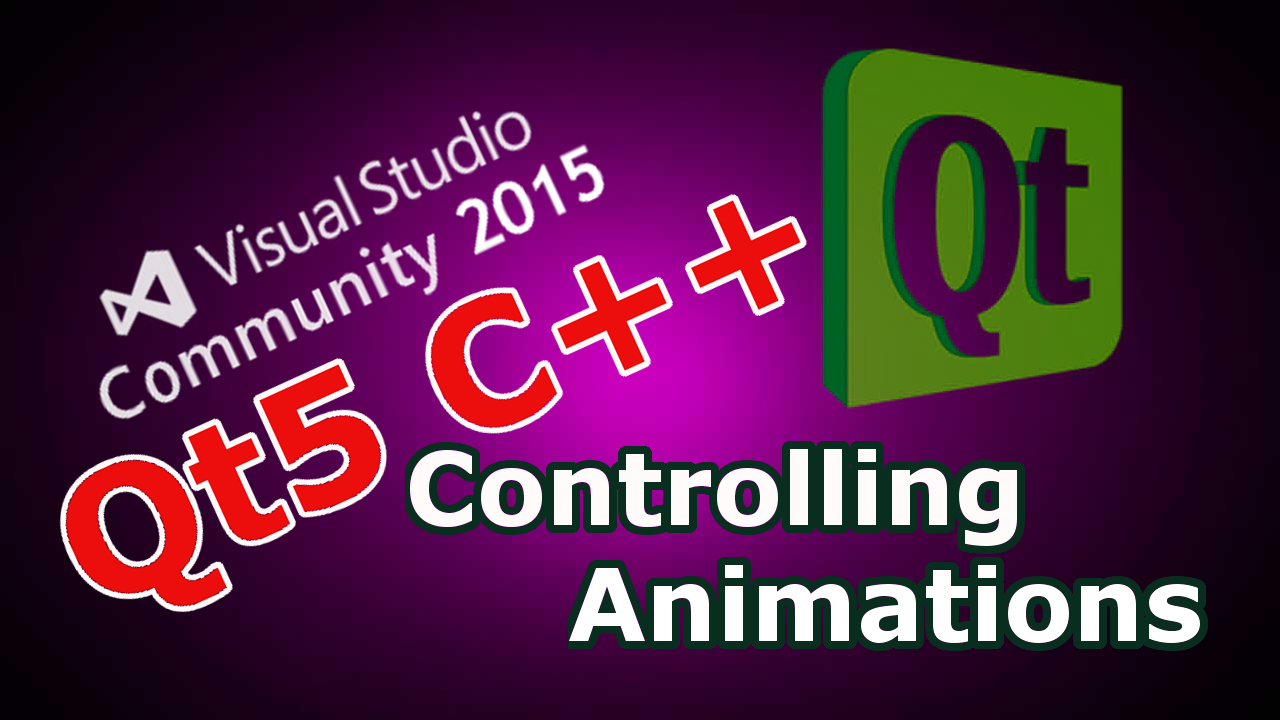
…because the slot will be expecting a QObject
that the signal will not send. This connection will report a runtime error.
Note that signal and slot arguments are not checked by the compiler when using this connect()
overload.
Advanced Signals and Slots Usage¶
For cases where you may require information on the sender of the signal, Qt provides the sender()
function, which returns a pointer to the object that sent the signal.
Lambda expressions are a convenient way to pass custom arguments to a slot:
Qt Signal Slot Connect
Using Qt with 3rd Party Signals and Slots¶
It is possible to use Qt with a 3rd party signal/slot mechanism. You can even use both mechanisms in the same project. Just add the following line to your qmake project (.pro) file.
It tells Qt not to define the moc keywords signals
, slots
, and emit
, because these names will be used by a 3rd party library, e.g. Boost. Then to continue using Qt signals and slots with the no_keywords
flag, simply replace all uses of the Qt moc keywords in your sources with the corresponding Qt macros Q_SIGNALS
(or Q_SIGNAL
), Q_SLOTS
(or Q_SLOT
), and Q_EMIT
.
See also
QLCDNumber
connect()
Digital Clock ExampleTetrix ExampleMeta-Object SystemQt’s Property System
Qt Signal Slot Infinite Loop 3
© 2019 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.